Model Deployment and Serving
Model Integration with App/API -
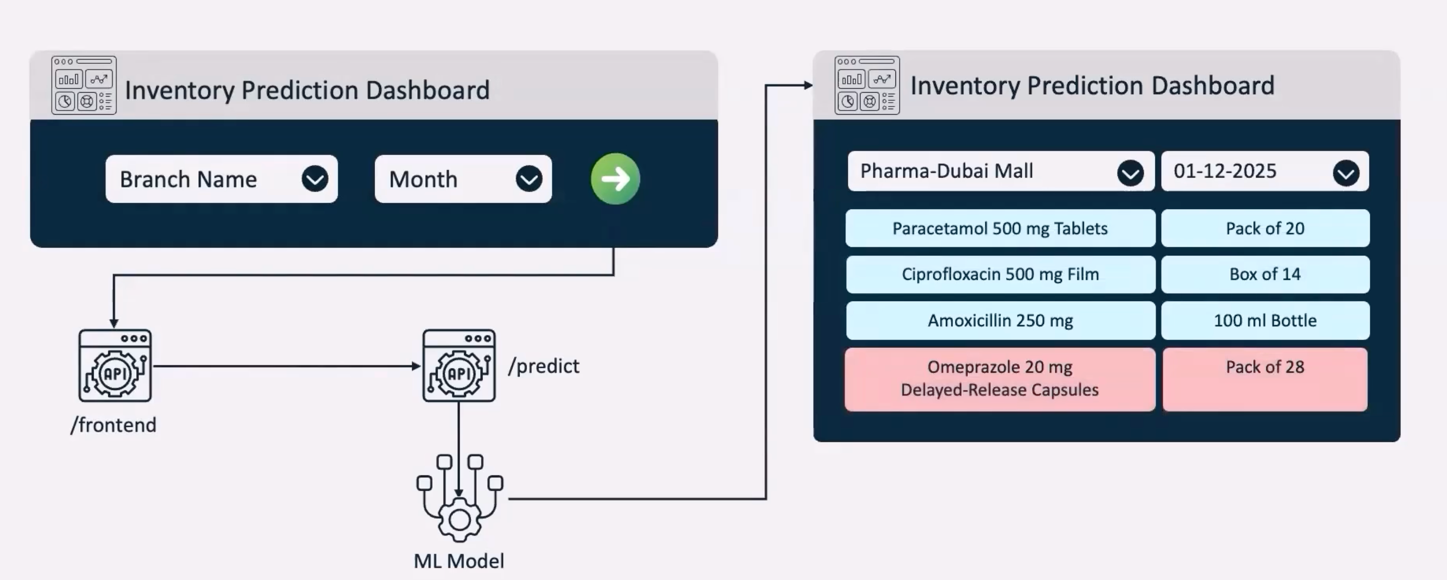
Initial Demo Script
1Model Deployment and Serving
2
3Once the Model Development and Experimentation is done...
4
5Integrate ML model with the applications API Gateway.
MLModel API Microservice -
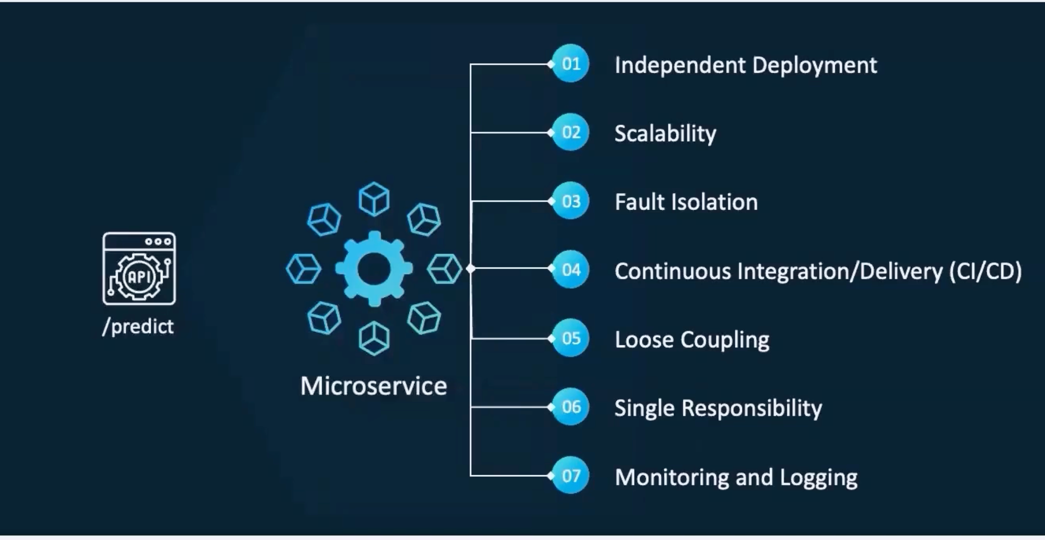
Deploy ML Model API
1Deploy ML model with an micro-service architecture
2
3/predict api contains a ML-Model and will be deployed as micro-service.. appliations /frontend api going to call this /predict API with parameters like branch_name and date :)
Online Prediction -
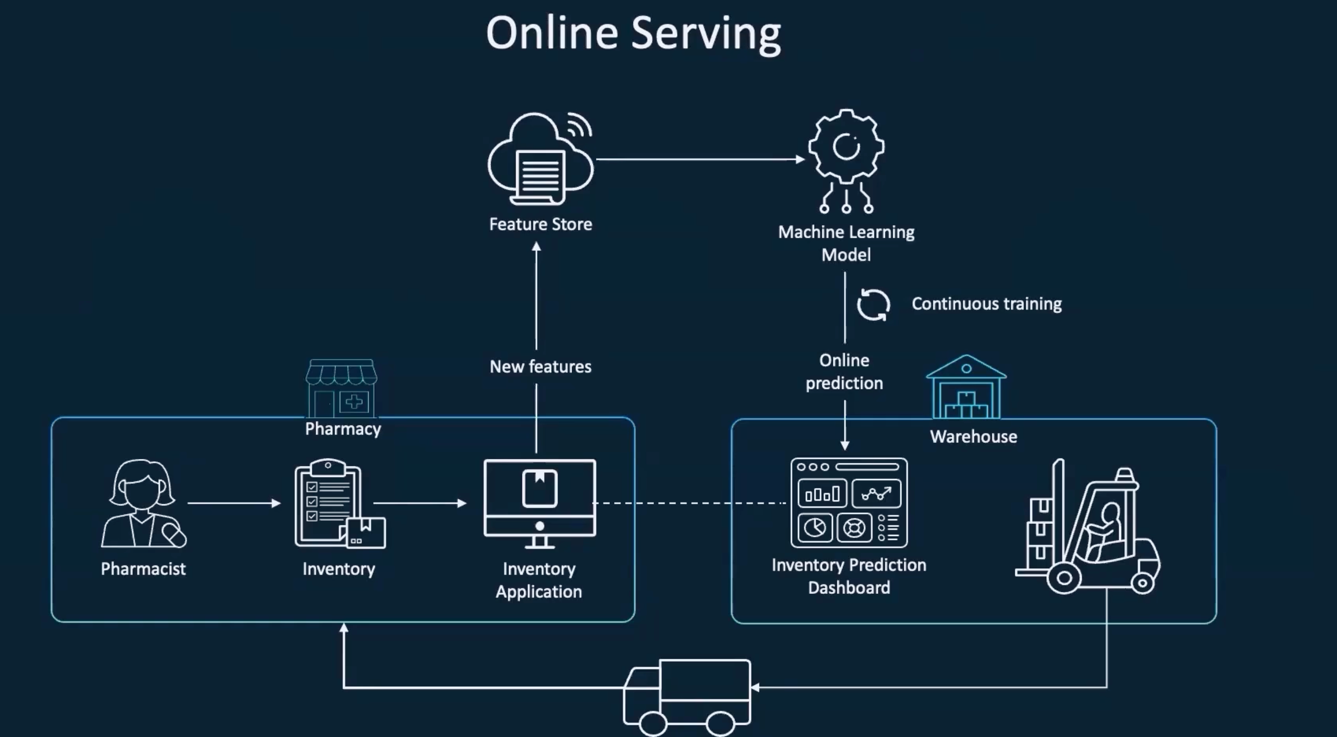
Model Drift & Offline/Online Serving
1When the model is ready in production , it is needed to be updated with new learning everytime.
2
3Consistently monitoring and retraing the model is necessary for the accurate prediction on the latest data.
4
5
6Online Serving:
7------------------->
8Whenever we get the new data(new feature) these data are getting updated to the feature-store, so based on this new input, Machine-Learning model continuously gets trained,This is called an online-prediction
9
10In the offline serving the new data getting updated to the feature store, but the machine learning model itself is getting trained in Batch Manner.
Offline Prediction -
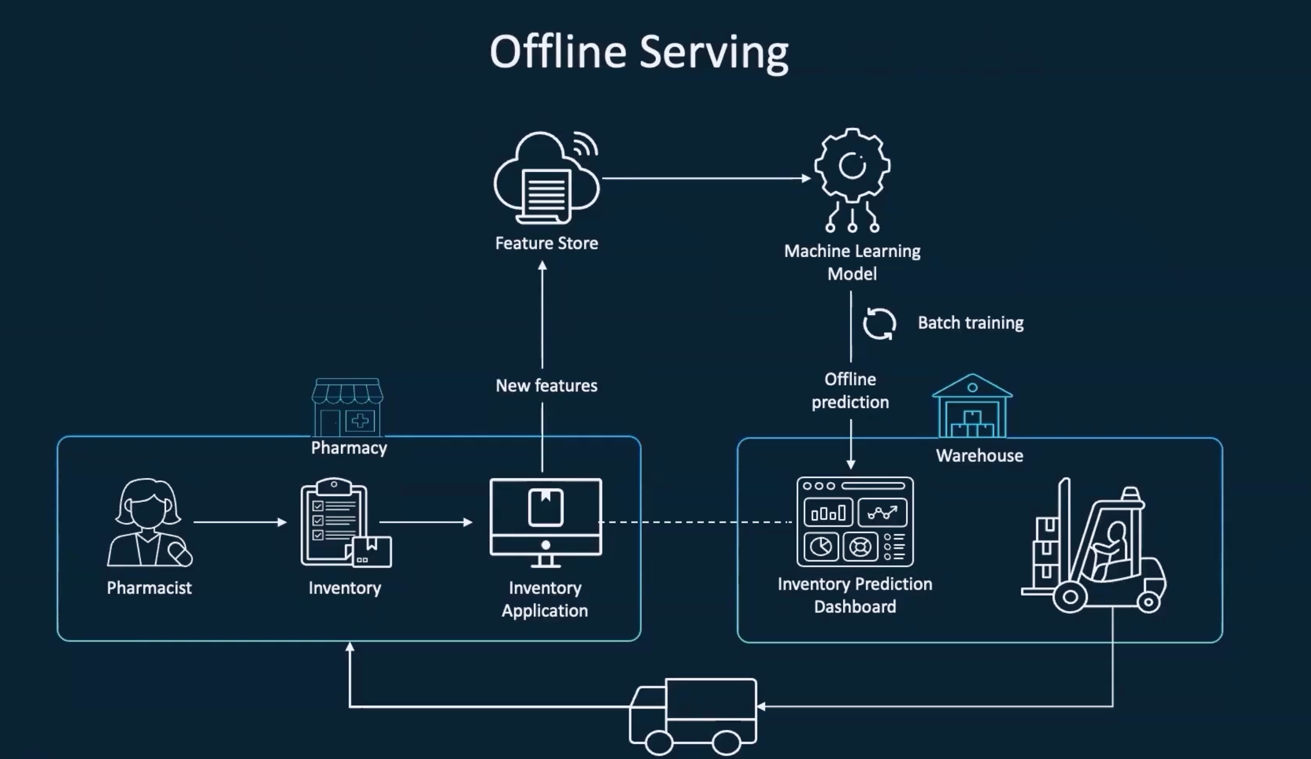
Model Serving Tools -
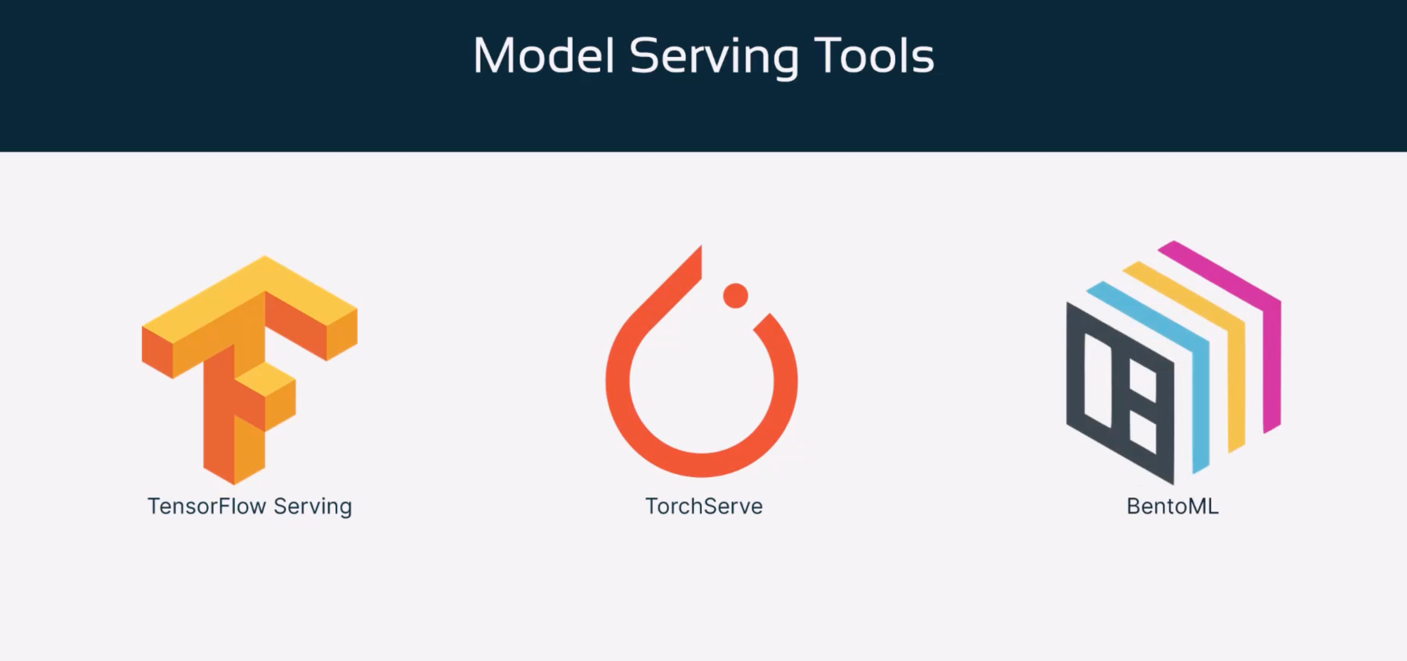
Simple ML Model Serving Architecture -
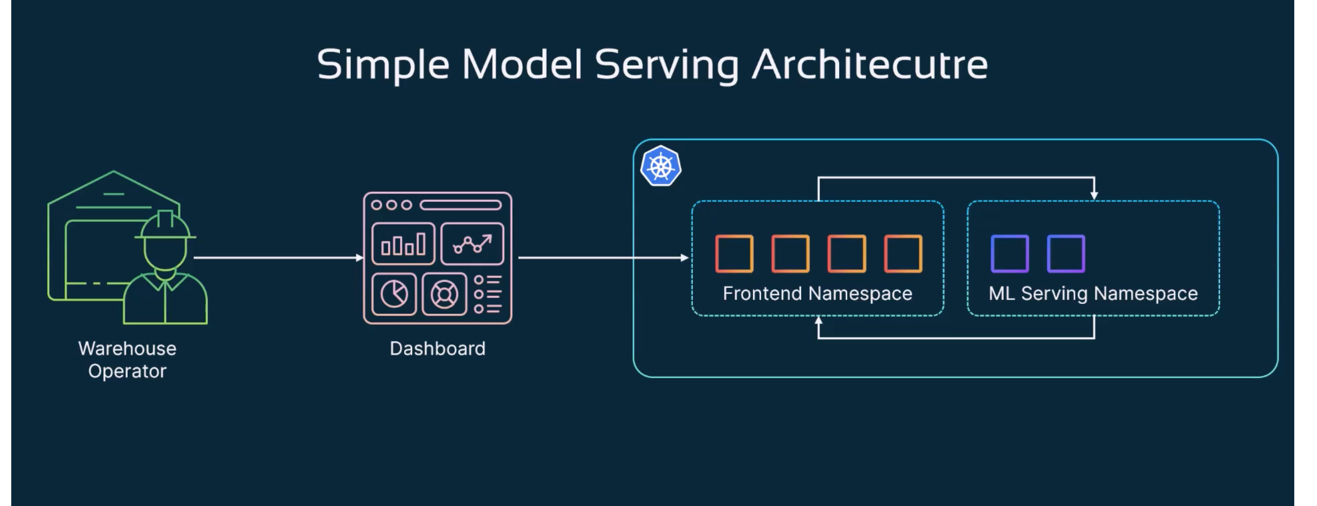
Script - Model Serving Tools
1Powerful Model Serving, Model deployments Frameworks
2
31. TensorFlow serving
42. PyTorch (TorchServe)
53. BentoML
6
7
8If we are working on tensorflow computer vision model that needs to process the thousands of image per second, Tensorflow serving is the goto choice.
9
10For PyTorch NLP models requiring A/B testing capabilities, ofcourse TorchServe is a goto choice - We can easily integrate TorchServe with Amazon Sagemaker or kubernetes for automated deployments.
11
12For teams needing Framework-agnostic solution with robust MLOps integration, BentoML fits perfectly.
Simple ML Model Serving Architecture v1 -
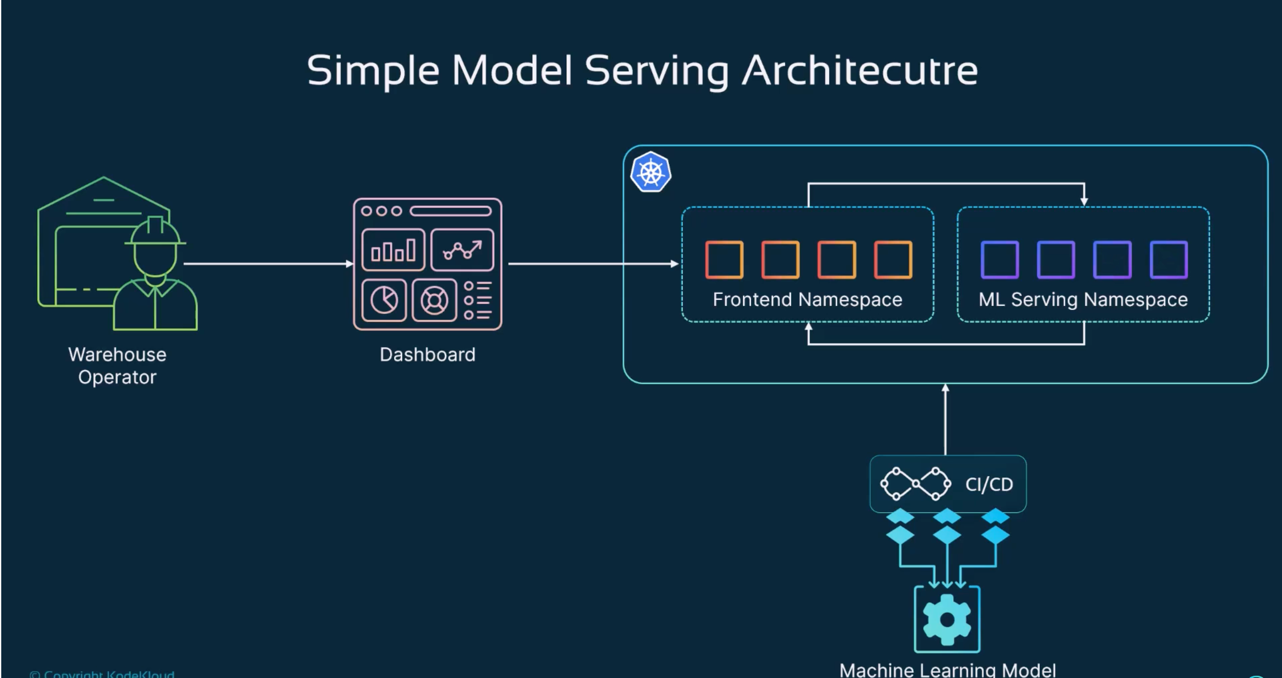
Model Service Code (BentoML)
Model Service V1
1import bentoml
2from bentoml.io import JSON
3from pydantic import BaseModel
4
5# Load the model
6model_ref = bentoml.sklearn.get("house_price_model:latest")
7model_runner = model_ref.to_runner()
8
9# Define the service
10svc = bentoml.Service("house_price_predictor", runners=[model_runner])
11
12# Input schema
13class HouseInput(BaseModel):
14 square_footage: float
15 num_rooms: int
16
17# API for prediction
18@svc.api(input=JSON(pydantic_model=HouseInput), output=JSON())
19async def predict_house_price(data: HouseInput):
20 input_data = [[data.square_footage, data.num_rooms]]
21 prediction = await model_runner.predict.async_run(input_data)
22 return {"predicted_price": prediction[0]}
Model Service V2
1import bentoml
2from bentoml.io import JSON
3from pydantic import BaseModel
4
5# Load the model
6model_ref = bentoml.sklearn.get("house_price_model_v2:latest")
7model_runner = model_ref.to_runner()
8
9# Define the service
10svc = bentoml.Service("house_price_predictor_v2", runners=[model_runner])
11
12# Input schema
13class HouseInput(BaseModel):
14 square_footage: float
15 num_rooms: int
16 num_bathrooms: int
17 house_age: int
18 distance_to_city_center: float
19 has_garage: int
20 has_garden: int
21 crime_rate: float
22 avg_school_rating: float
23 country: str
24
25# API for prediction
26@svc.api(input=JSON(pydantic_model=HouseInput), output=JSON())
27async def predict_house_price(data: HouseInput):
28 # One-hot encoding for the country
29 country_encoded = [0, 0, 0] # Default for ['Canada', 'Germany', 'UK']
30 if data.country == "Canada":
31 country_encoded[0] = 1
32 elif data.country == "Germany":
33 country_encoded[1] = 1
34 elif data.country == "UK":
35 country_encoded[2] = 1
36
37 input_data = [[
38 data.square_footage, data.num_rooms, data.num_bathrooms, data.house_age,
39 data.distance_to_city_center, data.has_garage, data.has_garden,
40 data.crime_rate, data.avg_school_rating
41 ] + country_encoded]
42
43 prediction = await model_runner.predict.async_run(input_data)
44 return {"predicted_price": prediction[0]}
Model Service V3
1import bentoml
2from bentoml.io import JSON
3from pydantic import BaseModel
4
5# Load the v1 and v2 models
6model_v1_ref = bentoml.sklearn.get("house_price_model:latest")
7model_v2_ref = bentoml.sklearn.get("house_price_model_v2:latest")
8model_v1_runner = model_v1_ref.to_runner()
9model_v2_runner = model_v2_ref.to_runner()
10
11# Define the service with both runners
12svc = bentoml.Service("house_price_predictor", runners=[model_v1_runner, model_v2_runner])
13
14# Input schema for V1 (simpler model)
15class HouseInputV1(BaseModel):
16 square_footage: float
17 num_rooms: int
18
19# Input schema for V2 (expanded model)
20class HouseInputV2(BaseModel):
21 square_footage: float
22 num_rooms: int
23 num_bathrooms: int
24 house_age: int
25 distance_to_city_center: float
26 has_garage: int
27 has_garden: int
28 crime_rate: float
29 avg_school_rating: float
30 country: str
31
32# API for V1 model prediction
33@svc.api(input=JSON(pydantic_model=HouseInputV1), output=JSON(), route="/predict_house_price_v1")
34async def predict_house_price_v1(data: HouseInputV1):
35 input_data = [[data.square_footage, data.num_rooms]]
36 prediction = await model_v1_runner.predict.async_run(input_data)
37 return {"predicted_price_v1": prediction[0]}
38
39# API for V2 model prediction
40@svc.api(input=JSON(pydantic_model=HouseInputV2), output=JSON(), route="/predict_house_price_v2")
41async def predict_house_price_v2(data: HouseInputV2):
42 # One-hot encoding for the country
43 country_encoded = [0, 0, 0] # Default for ['Canada', 'Germany', 'UK']
44 if data.country == "Canada":
45 country_encoded[0] = 1
46 elif data.country == "Germany":
47 country_encoded[1] = 1
48 elif data.country == "UK":
49 country_encoded[2] = 1
50
51 input_data = [[
52 data.square_footage, data.num_rooms, data.num_bathrooms, data.house_age,
53 data.distance_to_city_center, data.has_garage, data.has_garden,
54 data.crime_rate, data.avg_school_rating
55 ] + country_encoded]
56
57 prediction = await model_v2_runner.predict.async_run(input_data)
58 return {"predicted_price_v2": prediction[0]}
Model Training V1
1import pandas as pd
2from sklearn.linear_model import LinearRegression
3from sklearn.model_selection import train_test_split
4import bentoml
5
6# Generate synthetic data for house price prediction
7def generate_data():
8 data = {
9 'square_footage': [1000, 1500, 1800, 2000, 2300, 2500, 2700, 3000, 3200, 3500],
10 'num_rooms': [3, 4, 4, 5, 5, 6, 6, 7, 7, 8],
11 'price': [200000, 250000, 280000, 310000, 340000, 370000, 400000, 430000, 460000, 500000]
12 }
13 return pd.DataFrame(data)
14
15# Load the data
16df = generate_data()
17
18# Features and target
19X = df[['square_footage', 'num_rooms']]
20y = df['price']
21
22# Split the data
23X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
24
25# Train the model
26model = LinearRegression()
27model.fit(X_train, y_train)
28
29# Save the model with BentoML
30bentoml.sklearn.save_model("house_price_model", model)
Model Training V2
1import pandas as pd
2from sklearn.linear_model import LinearRegression
3from sklearn.model_selection import train_test_split
4import bentoml
5
6# Generate synthetic data for house price prediction with 10 features
7def generate_data():
8 data = {
9 'square_footage': [1000, 1500, 1800, 2000, 2300, 2500, 2700, 3000, 3200, 3500],
10 'num_rooms': [3, 4, 4, 5, 5, 6, 6, 7, 7, 8],
11 'num_bathrooms': [1, 2, 2, 2, 3, 3, 3, 4, 4, 4],
12 'house_age': [10, 5, 15, 20, 8, 12, 7, 3, 25, 30],
13 'distance_to_city_center': [10, 8, 12, 5, 15, 6, 20, 2, 18, 25],
14 'has_garage': [1, 1, 0, 1, 0, 1, 1, 1, 0, 1],
15 'has_garden': [1, 1, 1, 0, 1, 0, 0, 1, 1, 0],
16 'crime_rate': [0.3, 0.2, 0.5, 0.1, 0.4, 0.3, 0.6, 0.1, 0.7, 0.8],
17 'avg_school_rating': [8, 9, 7, 8, 6, 7, 5, 9, 4, 3],
18 'country': ['USA', 'USA', 'USA', 'Canada', 'Canada', 'Canada', 'UK', 'UK', 'Germany', 'Germany'],
19 'price': [200000, 250000, 280000, 310000, 340000, 370000, 400000, 430000, 460000, 500000]
20 }
21 return pd.DataFrame(data)
22
23# Load the data
24df = generate_data()
25
26# One-hot encode categorical features like 'country'
27df = pd.get_dummies(df, columns=['country'], drop_first=True)
28
29# Features and target
30X = df.drop(columns=['price'])
31y = df['price']
32
33# Split the data
34X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
35
36# Train the model
37model = LinearRegression()
38model.fit(X_train, y_train)
39
40# Save the model with BentoML
41bentoml.sklearn.save_model("house_price_model_v2", model)
README - BentoML Service
1# House Price Prediction with BentoML
2
3This project demonstrates how to build, train, and deploy a house price prediction model using Python, BentoML, and various machine learning libraries. Follow the steps below to set up the environment, train the model, serve it using BentoML, and make predictions via API requests.
4
5## Table of Contents
6
7- [House Price Prediction with BentoML](#house-price-prediction-with-bentoml)
8 - [Table of Contents](#table-of-contents)
9 - [Prerequisites](#prerequisites)
10 - [Setup](#setup)
11 - [Training the Model](#training-the-model)
12 - [Version 1](#version-1)
13 - [Serving the Model](#serving-the-model)
14 - [Version 1](#version-1-1)
15 - [Version 2](#version-2)
16 - [Version 3](#version-3)
17 - [Versioning](#versioning)
18 - [Cleanup](#cleanup)
19
20## Prerequisites
21
22Ensure you have the following installed on your local machine:
23
24- **Python 3.7+**
25- **pip**
26- **Git** (optional, for version control)
27
28## Setup
29
301. **Create a Virtual Environment**
31
32 ```bash
33 python3 -m venv bentoml-env
34 ```
35
362. **Activate the Virtual Environment**
37
38 ```bash
39 source bentoml-env/bin/activate
40 ```
41
423. **Install Required Packages**
43
44 ```bash
45 pip3 install bentoml scikit-learn pandas
46 ```
47
484. **Navigate to the Project Directory**
49
50 ```bash
51 cd 04-bentoml
52 ```
53
54## Training the Model
55
56### Version 1
57
581. **Train the Initial Model**
59
60 ```bash
61 python3 model_train_v1.py
62 ```
63
642. **List Available BentoML Models**
65
66 ```bash
67 bentoml models list
68 ```
69
70## Serving the Model
71
72### Version 1
73
741. **Serve the Model with BentoML**
75
76 ```bash
77 bentoml serve model_service_v1.py --reload
78 ```
79
802. **Make a Prediction Request**
81
82 Open a new terminal window/tab, activate the virtual environment, navigate to the project directory, and run:
83
84 ```bash
85 curl -X POST "http://127.0.0.1:3000/predict_house_price" \
86 -H "Content-Type: application/json" \
87 -d '{"square_footage": 2500, "num_rooms": 5}'
88 ```
89
90### Version 2
91
921. **Train the Enhanced Model**
93
94 ```bash
95 python3 model_train_v2.py
96 ```
97
982. **Serve the Enhanced Model**
99
100 ```bash
101 bentoml serve model_service_v2.py --reload
102 ```
103
1043. **Make a Detailed Prediction Request**
105
106 ```bash
107 curl -X POST "http://127.0.0.1:3000/predict_house_price" \
108 -H "Content-Type: application/json" \
109 -d '{
110 "square_footage": 2500,
111 "num_rooms": 5,
112 "num_bathrooms": 3,
113 "house_age": 10,
114 "distance_to_city_center": 8,
115 "has_garage": 1,
116 "has_garden": 1,
117 "crime_rate": 0.2,
118 "avg_school_rating": 8,
119 "country": "Germany"
120 }'
121 ```
122
123### Version 3
124
1251. **Serve Additional Model Versions**
126
127 ```bash
128 bentoml serve model_service_v3.py --reload
129 ```
130
1312. **Make Prediction Requests to Specific Model Versions**
132
133 - **Version 1 Endpoint**
134
135 ```bash
136 curl -X POST "http://127.0.0.1:3000/predict_house_price_v1" \
137 -H "Content-Type: application/json" \
138 -d '{"square_footage": 2500, "num_rooms": 5}'
139 ```
140
141 - **Version 2 Endpoint**
142
143 ```bash
144 curl -X POST "http://127.0.0.1:3000/predict_house_price_v2" \
145 -H "Content-Type: application/json" \
146 -d '{
147 "square_footage": 2500,
148 "num_rooms": 5,
149 "num_bathrooms": 3,
150 "house_age": 10,
151 "distance_to_city_center": 8,
152 "has_garage": 1,
153 "has_garden": 1,
154 "crime_rate": 0.2,
155 "avg_school_rating": 8,
156 "country": "Germany"
157 }'
158 ```
159
160## Versioning
161
162Each version of the model and its corresponding service script allows for iterative improvements and testing. Ensure that you train and serve the appropriate version based on your requirements.
163
164## Cleanup
165
166To deactivate the virtual environment and clean up your terminal:
167
1681. **Deactivate the Virtual Environment**
169
170 ```bash
171 deactivate
172 ```
173
1742. **Clear the Terminal (Optional)**
175
176 ```bash
177 clear
178 ```
179
180---
BentoML Prediction Service -
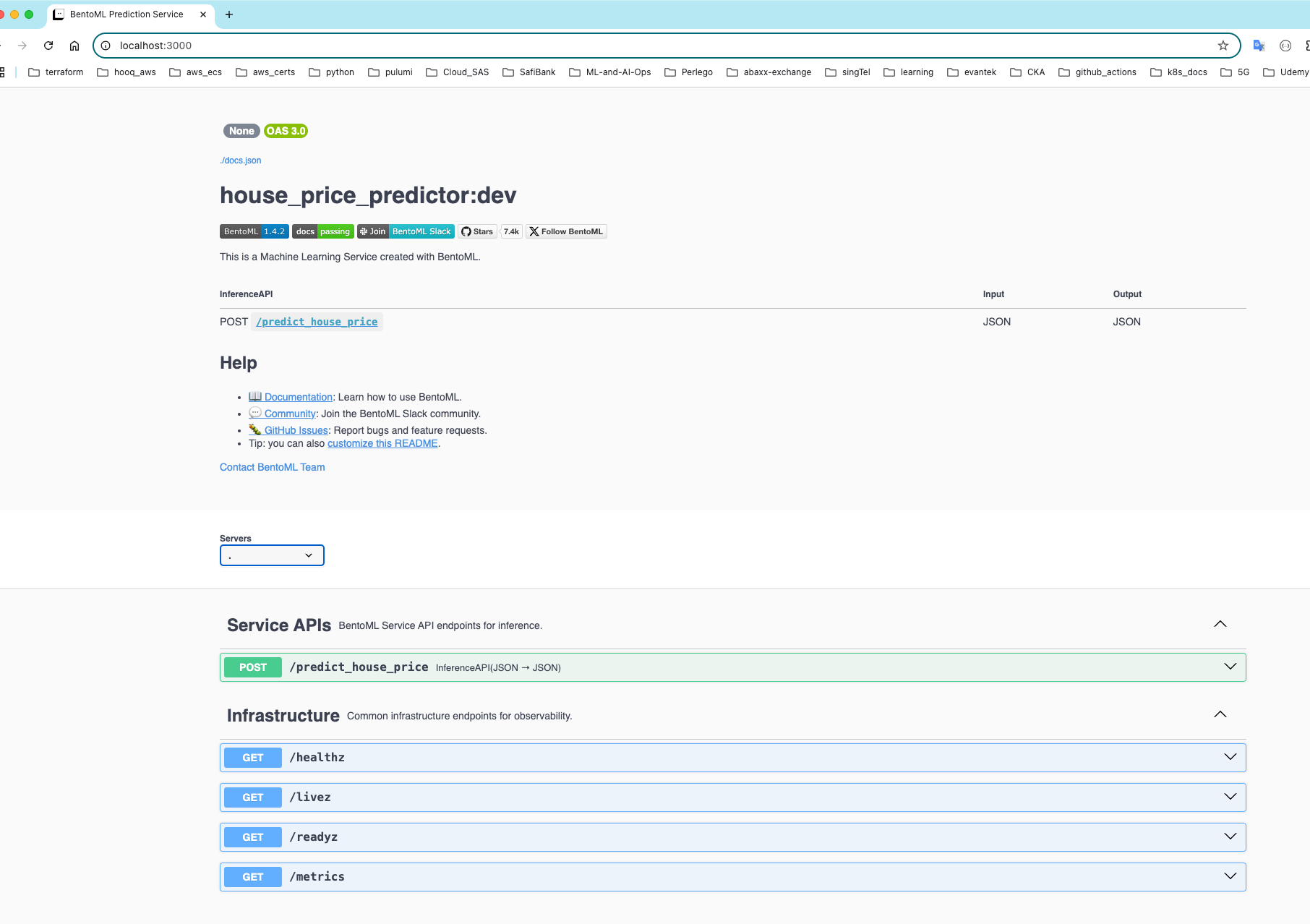
House Price Prediction using BentoML -
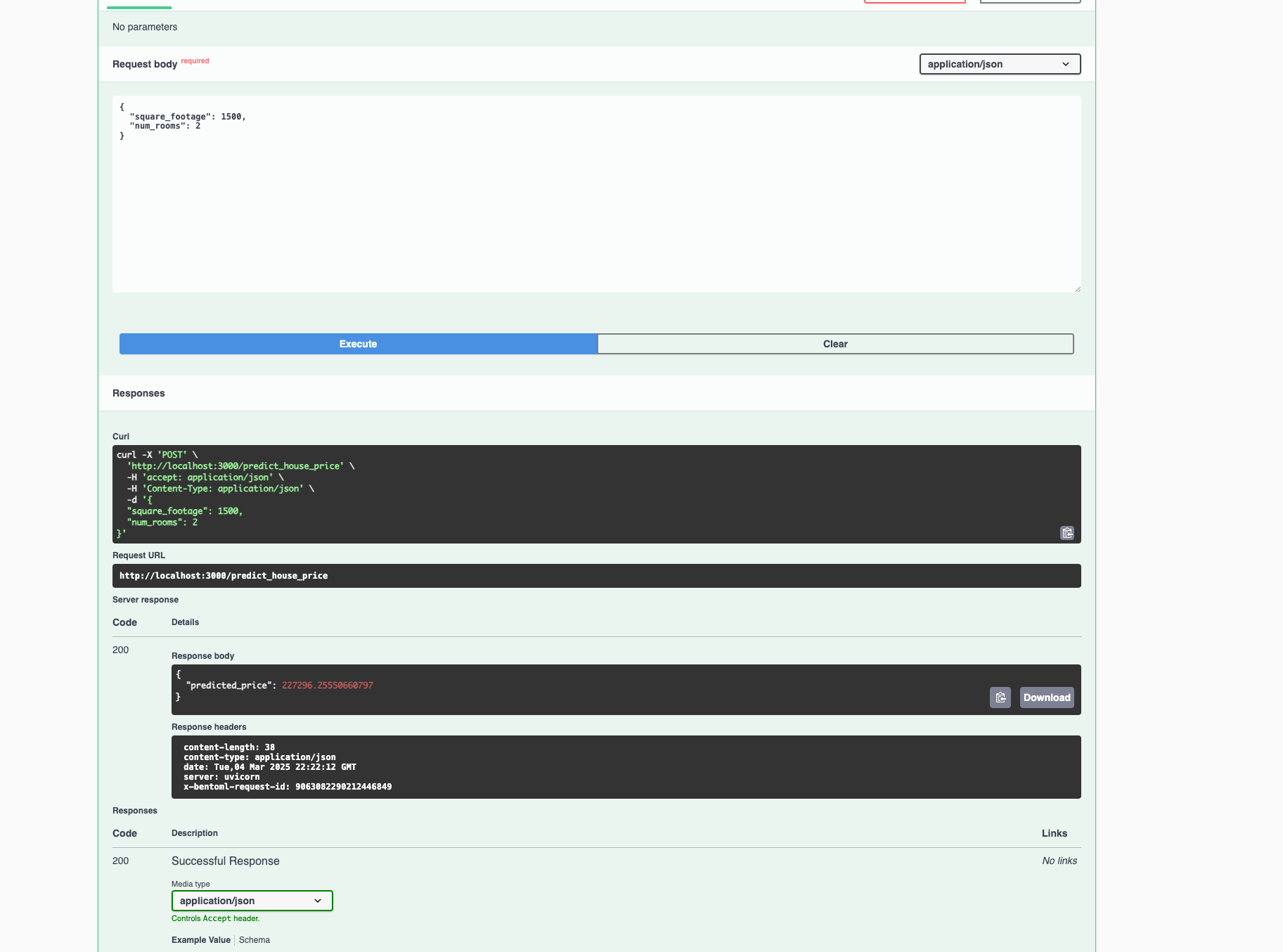
Serve Multiple ML Models with BentoML -
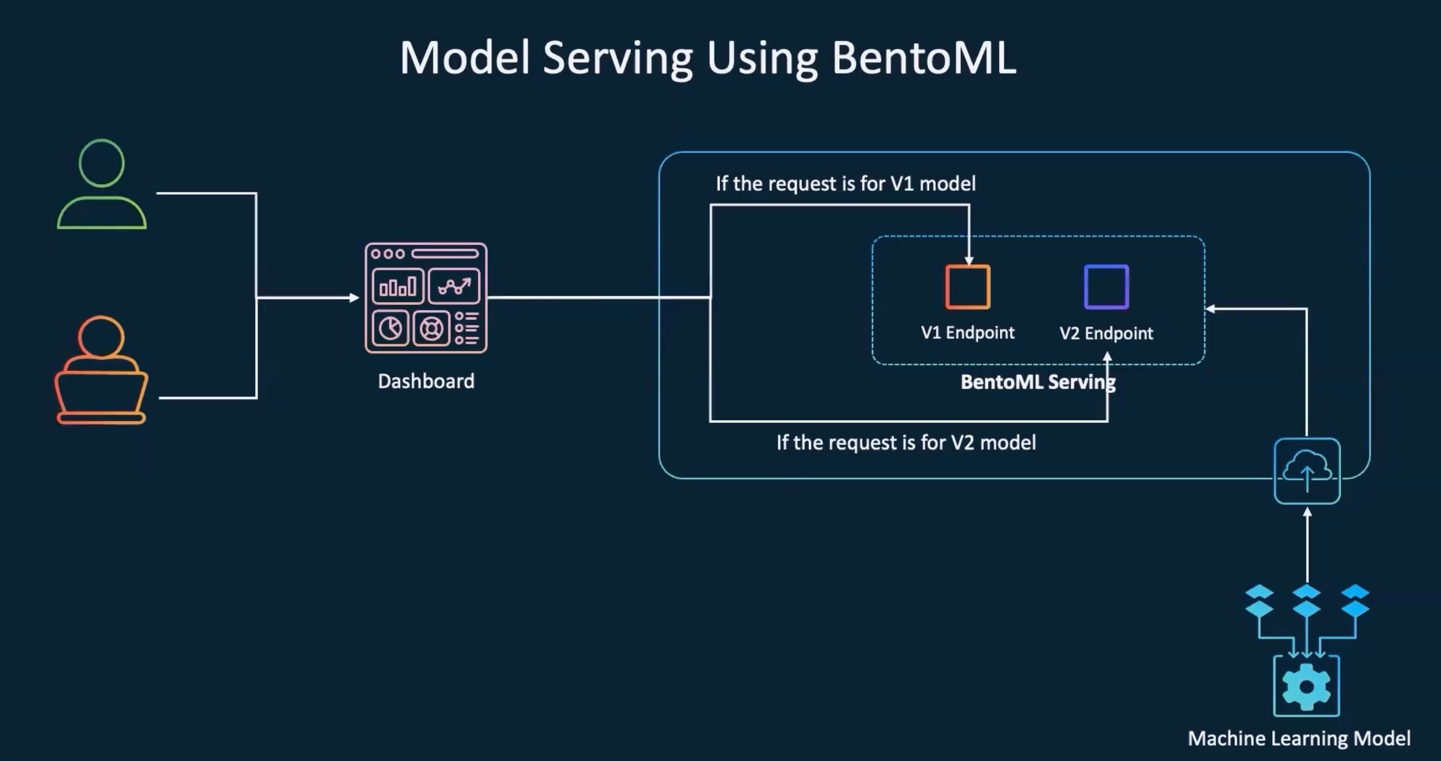
Deploy Model in BentoML
1BentoML Serving platform hoting our ML model that is stored in the artifact registry, either mlflow or BentoML repository itself.
2
3
4(.venv) bharathkumardasaraju@1.demo-model-train-service-BentoML$ python3.12 ./model_train_v1.py
5(.venv) bharathkumardasaraju@1.demo-model-train-service-BentoML$ bentoml models list
6 Tag Module Size Creation Time
7 house_price_model:ymkym6xziwjagprc bentoml.sklearn 1.23 KiB 2025-03-05 06:12:29
8(.venv) bharathkumardasaraju@1.demo-model-train-service-BentoML$
9
10Using the above model we will be able to create an API endpoint that could be called by other micro-services - by using BentoML.
11
12
13(.venv) bharathkumardasaraju@1.demo-model-train-service-BentoML$ bentoml serve ./model_service_v1.py --reload
14/Users/bharathkumardasaraju/external/learn-ml-ops/.venv/lib/python3.12/site-packages/bentoml/io.py:7: BentoMLDeprecationWarning: `bentoml.io` is deprecated since BentoML v1.4 and will be removed in a future version. Please upgrade to new style IO types instead.
15 warn_deprecated(
16/Users/bharathkumardasaraju/external/learn-ml-ops/.venv/lib/python3.12/site-packages/bentoml/_internal/models/model.py:367: BentoMLDeprecationWarning: `get_runnable` is deprecated since BentoML v1.4 and will be removed in a future version. Use `get_service` instead.
17 self._runnable = self.info.imported_module.get_runnable(self)
18/Users/bharathkumardasaraju/external/learn-ml-ops/.venv/lib/python3.12/site-packages/bentoml/_internal/models/model.py:354: BentoMLDeprecationWarning: `Runner` is deprecated since BentoML v1.4 and will be removed in a future version. Please upgrade to new style services.
19 return Runner(
20/Users/bharathkumardasaraju/external/learn-ml-ops/5.Model-Deployment-and-Serving/1.demo-model-train-service-BentoML/model_service_v1.py:10: BentoMLDeprecationWarning: `bentoml.Service` is deprecated since BentoML v1.4 and will be removed in a future version. Please upgrade to @bentoml.service().
21 svc = bentoml.Service("house_price_predictor", runners=[model_runner])
222025-03-05T06:18:29+0800 [INFO] [cli] Environ for worker 0: set CPU thread count to 8
232025-03-05T06:18:29+0800 [INFO] [cli] Prometheus metrics for HTTP BentoServer from "./model_service_v1.py" can be accessed at http://localhost:3000/metrics.
242025-03-05T06:18:29+0800 [INFO] [cli] Starting production HTTP BentoServer from "./model_service_v1.py" listening on http://0.0.0.0:3000 (Press CTRL+C to quit)
252025-03-05T06:19:30+0800 [INFO] [api_server:house_price_predictor:7] 127.0.0.1:62195 (scheme=http,method=GET,path=/,type=,length=) (status=200,type=text/html; charset=utf-8,length=2945) 0.909ms (trace=8978d0eb6894190c2a47e763fc209588,span=f2c5f61991d01d9a,sampled=0,service.name=house_price_predictor)
262025-03-05T06:19:30+0800 [INFO] [api_server:house_price_predictor:7] 127.0.0.1:62195 (scheme=http,method=GET,path=/static_content/swagger-ui.css,type=,length=) (status=200,type=text/css; charset=utf-8,length=152059) 12.396ms (trace=9d4ac53b151f2a6dcd079302a74fa7b1,span=bf82a11a273a3ae4,sampled=0,service.name=house_price_predictor)
272025-03-05T06:19:30+0800 [INFO] [api_server:house_price_predictor:8] 127.0.0.1:62196 (scheme=http,method=GET,path=/static_content/index.css,type=,length=) (status=200,type=text/css; charset=utf-8,length=1127) 8.001ms (trace=63b573791ba86aa360158a850261428a,span=2601acd02c445a68,sampled=0,service.name=house_price_predictor)
282025-03-05T06:19:30+0800 [INFO] [api_server:house_price_predictor:6] 127.0.0.1:62202 (scheme=http,method=GET,path=/static_content/swagger-ui-standalone-preset.js,type=,length=) (status=200,type=text/javascript; charset=utf-8,length=230777) 13.038ms (trace=ec1773c8e2eab4e24761d24cecbe936a,span=521abcf701ace710,sampled=0,service.name=house_price_predictor)
292025-03-05T06:19:30+0800 [INFO] [api_server:house_price_predictor:1] 127.0.0.1:62203 (scheme=http,method=GET,path=/static_content/swagger-initializer.js,type=,length=) (status=200,type=text/javascript; charset=utf-8,length=331) 9.868ms (trace=a5872a906bbff9ea1734e5b38c0d80a6,span=5e48f507a62e7f52,sampled=0,service.name=house_price_predictor)
302025-03-05T06:19:30+0800 [INFO] [api_server:house_price_predictor:4] 127.0.0.1:62201 (scheme=http,method=GET,path=/static_content/swagger-ui-bundle.js,type=,length=) (status=200,type=text/javascript; charset=utf-8,length=1415333) 33.879ms (trace=e6c2a6d776fda606483116ab01f7e34d,span=aac2d6eef78370e3,sampled=0,service.name=house_price_predictor)
312025-03-05T06:19:31+0800 [INFO] [api_server:house_price_predictor:4] 127.0.0.1:62201 (scheme=http,method=GET,path=/docs.json,type=,length=) (status=200,type=application/json,length=5098) 22.405ms (trace=7acffd095d6f3d7e9995185f1a13e7f5,span=48b17fce66c2c6de,sampled=0,service.name=house_price_predictor)
32
33
34
35
36bharathkumardasaraju@elastic-snapshot$ curl -X POST "http://127.0.0.1:3000/predict_house_price" \
37 -H "Content-Type: application/json" \
38 -d '{"square_footage": 2500, "num_rooms": 5}'
39{"predicted_price":360974.66960352426}%
40bharathkumardasaraju@elastic-snapshot$
41
42
43
44
45
46(.venv) bharathkumardasaraju@1.demo-model-train-service-BentoML$ python3.12 ./model_train_v2.py
47(.venv) bharathkumardasaraju@1.demo-model-train-service-BentoML$ bentoml models list
48 Tag Module Size Creation Time
49 house_price_model_v2:v2nt7nxzjcngcprc bentoml.sklearn 1.56 KiB 2025-03-05 06:33:23
50 house_price_model:ymkym6xziwjagprc bentoml.sklearn 1.23 KiB 2025-03-05 06:12:29
51(.venv) bharathkumardasaraju@1.demo-model-train-service-BentoML$
52
53(.venv) bharathkumardasaraju@1.demo-model-train-service-BentoML$ bentoml serve ./model_service_v2.py --reload
54/Users/bharathkumardasaraju/external/learn-ml-ops/.venv/lib/python3.12/site-packages/bentoml/io.py:7: BentoMLDeprecationWarning: `bentoml.io` is deprecated since BentoML v1.4 and will be removed in a future version. Please upgrade to new style IO types instead.
55 warn_deprecated(
56/Users/bharathkumardasaraju/external/learn-ml-ops/.venv/lib/python3.12/site-packages/bentoml/_internal/models/model.py:367: BentoMLDeprecationWarning: `get_runnable` is deprecated since BentoML v1.4 and will be removed in a future version. Use `get_service` instead.
57 self._runnable = self.info.imported_module.get_runnable(self)
58/Users/bharathkumardasaraju/external/learn-ml-ops/.venv/lib/python3.12/site-packages/bentoml/_internal/models/model.py:354: BentoMLDeprecationWarning: `Runner` is deprecated since BentoML v1.4 and will be removed in a future version. Please upgrade to new style services.
59 return Runner(
60/Users/bharathkumardasaraju/external/learn-ml-ops/5.Model-Deployment-and-Serving/1.demo-model-train-service-BentoML/model_service_v2.py:10: BentoMLDeprecationWarning: `bentoml.Service` is deprecated since BentoML v1.4 and will be removed in a future version. Please upgrade to @bentoml.service().
61 svc = bentoml.Service("house_price_predictor_v2", runners=[model_runner])
622025-03-05T06:34:32+0800 [INFO] [cli] Environ for worker 0: set CPU thread count to 8
632025-03-05T06:34:32+0800 [INFO] [cli] Prometheus metrics for HTTP BentoServer from "./model_service_v2.py" can be accessed at http://localhost:3000/metrics.
642025-03-05T06:34:33+0800 [INFO] [cli] Starting production HTTP BentoServer from "./model_service_v2.py" listening on http://0.0.0.0:3000 (Press CTRL+C to quit)
652025-03-05T06:35:30+0800 [INFO] [api_server:house_price_predictor_v2:2] 127.0.0.1:62779 (scheme=http,method=GET,path=/,type=,length=) (status=200,type=text/html; charset=utf-8,length=2945) 0.474ms (trace=18766c94f7b3d5856524c88cc3697727,span=7b3a19b7ab2d46ab,sampled=0,service.name=house_price_predictor_v2)
662025-03-05T06:35:30+0800 [INFO] [api_server:house_price_predictor_v2:2] 127.0.0.1:62779 (scheme=http,method=GET,path=/static_content/swagger-ui.css,type=,length=) (status=304,type=,length=) 16.521ms (trace=51fd56906dcef284e0948367f1418b7e,span=a298b093920184db,sampled=0,service.name=house_price_predictor_v2)
672025-03-05T06:35:30+0800 [INFO] [api_server:house_price_predictor_v2:2] 127.0.0.1:62784 (scheme=http,method=GET,path=/static_content/swagger-ui-bundle.js,type=,length=) (status=304,type=,length=) 6.798ms (trace=f552e21138fc3b9ac26eec200945d383,span=443e7c14234c6154,sampled=0,service.name=house_price_predictor_v2)
682025-03-05T06:35:30+0800 [INFO] [api_server:house_price_predictor_v2:1] 127.0.0.1:62780 (scheme=http,method=GET,path=/static_content/index.css,type=,length=) (status=304,type=,length=) 12.268ms (trace=0cf5abcc5fe116498f3e63b2997fa462,span=4dc38d2677d16a7b,sampled=0,service.name=house_price_predictor_v2)
692025-03-05T06:35:30+0800 [INFO] [api_server:house_price_predictor_v2:3] 127.0.0.1:62787 (scheme=http,method=GET,path=/static_content/swagger-ui-standalone-preset.js,type=,length=) (status=304,type=,length=) 11.479ms (trace=654addd10925550a2ef15ea65f3e721c,span=d595036abc0dac06,sampled=0,service.name=house_price_predictor_v2)
70
71
72
73bharathkumardasaraju@elastic-snapshot$ curl -X POST "http://127.0.0.1:3000/predict_house_price" \
74 -H "Content-Type: application/json" \
75 -d '{
76 "square_footage": 2500,
77 "num_rooms": 5,
78 "num_bathrooms": 3,
79 "house_age": 10,
80 "distance_to_city_center": 8,
81 "has_garage": 1,
82 "has_garden": 1,
83 "crime_rate": 0.2,
84 "avg_school_rating": 8,
85 "country": "Germany"
86 }'
87{"predicted_price":366760.9746143092}%
88bharathkumardasaraju@elastic-snapshot$
89
90Serving with the multiple models using same BEntoMl Endpoint
91
92(.venv) bharathkumardasaraju@1.demo-model-train-service-BentoML$
93(.venv) bharathkumardasaraju@1.demo-model-train-service-BentoML$ bentoml serve ./model_service_v3.py --reload
94/Users/bharathkumardasaraju/external/learn-ml-ops/.venv/lib/python3.12/site-packages/bentoml/io.py:7: BentoMLDeprecationWarning: `bentoml.io` is deprecated since BentoML v1.4 and will be removed in a future version. Please upgrade to new style IO types instead.
95 warn_deprecated(
96/Users/bharathkumardasaraju/external/learn-ml-ops/.venv/lib/python3.12/site-packages/bentoml/_internal/models/model.py:367: BentoMLDeprecationWarning: `get_runnable` is deprecated since BentoML v1.4 and will be removed in a future version. Use `get_service` instead.
97 self._runnable = self.info.imported_module.get_runnable(self)
98/Users/bharathkumardasaraju/external/learn-ml-ops/.venv/lib/python3.12/site-packages/bentoml/_internal/models/model.py:354: BentoMLDeprecationWarning: `Runner` is deprecated since BentoML v1.4 and will be removed in a future version. Please upgrade to new style services.
99 return Runner(
100/Users/bharathkumardasaraju/external/learn-ml-ops/5.Model-Deployment-and-Serving/1.demo-model-train-service-BentoML/model_service_v3.py:12: BentoMLDeprecationWarning: `bentoml.Service` is deprecated since BentoML v1.4 and will be removed in a future version. Please upgrade to @bentoml.service().
101 svc = bentoml.Service("house_price_predictor", runners=[model_v1_runner, model_v2_runner])
1022025-03-05T06:41:59+0800 [INFO] [cli] Environ for worker 0: set CPU thread count to 8
1032025-03-05T06:41:59+0800 [INFO] [cli] Environ for worker 0: set CPU thread count to 8
1042025-03-05T06:41:59+0800 [INFO] [cli] Prometheus metrics for HTTP BentoServer from "./model_service_v3.py" can be accessed at http://localhost:3000/metrics.
1052025-03-05T06:42:00+0800 [INFO] [cli] Starting production HTTP BentoServer from "./model_service_v3.py" listening on http://0.0.0.0:3000 (Press CTRL+C to quit)
1062025-03-05T06:42:07+0800 [INFO] [api_server:house_price_predictor:7] 127.0.0.1:63078 (scheme=http,method=GET,path=/,type=,length=) (status=200,type=text/html; charset=utf-8,length=2945) 0.779ms (trace=b23311a398acc3be477b1101276a8583,span=d0fc8d1ab7f7600c,sampled=0,service.name=house_price_predictor)
1072025-03-05T06:42:07+0800 [INFO] [api_server:house_price_predictor:7] 127.0.0.1:63078 (scheme=http,method=GET,path=/static_content/index.css,type=,length=) (status=304,type=,length=) 21.008ms (trace=6d29c0223563a23297e9b2675f86160c,span=db54c42be948e88b,sampled=0,service.name=house_price_predictor)
1082025-03-05T06:42:07+0800 [INFO] [api_server:house_price_predictor:7] 127.0.0.1:63083 (scheme=http,method=GET,path=/static_content/swagger-ui-standalone-preset.js,type=,length=) (status=304,type=,length=) 10.899ms (trace=ff16ca522e266380de23129f7a8199db,span=1833c6e51ca2ad57,sampled=0,service.name=house_price_predictor)
1092025-03-05T06:42:07+0800 [INFO] [api_server:house_price_predictor:7] 127.0.0.1:63082 (scheme=http,method=GET,path=/static_content/swagger-ui.css,type=,length=) (status=304,type=,length=) 12.913ms (trace=d0a953aa19cfe839fcc2520c4978d5f9,span=859960399bf06a77,sampled=0,service.name=house_price_predictor)
1102025-03-05T06:42:07+0800 [INFO] [api_server:house_price_predictor:7] 127.0.0.1:63085 (scheme=http,method=GET,path=/static_content/swagger-initializer.js,type=,length=) (status=304,type=,length=) 15.737ms (trace=9ec080e1b6d8a03d2c278f7d565d1ac3,span=98bb93083fd15aee,sampled=0,service.name=house_price_predictor)
111
112
113
114bharathkumardasaraju@elastic-snapshot$ curl -X POST "http://127.0.0.1:3000/predict_house_price_v1" \
115 -H "Content-Type: application/json" \
116 -d '{"square_footage": 2500, "num_rooms": 5}'
117{"predicted_price_v1":360974.66960352426}%
118bharathkumardasaraju@elastic-snapshot$
119bharathkumardasaraju@elastic-snapshot$
120bharathkumardasaraju@elastic-snapshot$ curl -X POST "http://127.0.0.1:3000/predict_house_price_v2" \
121 -H "Content-Type: application/json" \
122 -d '{
123 "square_footage": 2500,
124 "num_rooms": 5,
125 "num_bathrooms": 3,
126 "house_age": 10,
127 "distance_to_city_center": 8,
128 "has_garage": 1,
129 "has_garden": 1,
130 "crime_rate": 0.2,
131 "avg_school_rating": 8,
132 "country": "Germany"
133 }'
134{"predicted_price_v2":366760.9746143092}%
135bharathkumardasaraju@elastic-snapshot$
ML Model Testing with BentoML
ML Model Testing Script
1root@controlplane ~ ➜ cd ~/lab/04-bentoml
2
3root@controlplane ~/lab/04-bentoml via 🐍 v3.12.3 ➜ python3 -m venv bentoml-env
4
5root@controlplane ~/lab/04-bentoml via 🐍 v3.12.3 ➜ source bentoml-env/bin/activate
6
7root@controlplane ~/lab/04-bentoml via 🐍 v3.12.3 (bentoml-env) ➜ pip3 install bentoml scikit-learn pandas
8Collecting bentoml
9 Using cached bentoml-1.4.2-py3-none-any.whl.metadata (16 kB)
10Collecting scikit-learn
11root@controlplane ~/lab/04-bentoml via 🐍 v3.12.3 (bentoml-env) ➜
12
13
14root@controlplane ~/lab/04-bentoml via 🐍 v3.12.3 (bentoml-env) ➜ python3 ./model_train_v1.py
15
16root@controlplane ~/lab/04-bentoml via 🐍 v3.12.3 (bentoml-env) ➜ bentoml models list
17 Tag Module Size Creation Time
18 house_price_model:zbsn3yhzjockdisu bentoml.sklearn 1.23 KiB 2025-03-04 17:55:35
19
20root@controlplane ~/lab/04-bentoml via 🐍 v3.12.3 (bentoml-env) ➜
21
22root@controlplane ~/lab/04-bentoml via 🐍 v3.12.3 (bentoml-env) ➜ bentoml serve model_service_v1.py --reload
23/root/lab/04-bentoml/bentoml-env/lib/python3.12/site-packages/bentoml/io.py:7: BentoMLDeprecationWarning: `bentoml.io` is deprecated since BentoML v1.4 and will be removed in a future version. Please upgrade to new style IO types instead.
24 warn_deprecated(
25/root/lab/04-bentoml/bentoml-env/lib/python3.12/site-packages/bentoml/_internal/models/model.py:367: BentoMLDeprecationWarning: `get_runnable` is deprecated since BentoML v1.4 and will be removed in a future version. Use `get_service` instead.
26 self._runnable = self.info.imported_module.get_runnable(self)
27/root/lab/04-bentoml/bentoml-env/lib/python3.12/site-packages/bentoml/_internal/models/model.py:354: BentoMLDeprecationWarning: `Runner` is deprecated since BentoML v1.4 and will be removed in a future version. Please upgrade to new style services.
28 return Runner(
29/root/lab/04-bentoml/model_service_v1.py:10: BentoMLDeprecationWarning: `bentoml.Service` is deprecated since BentoML v1.4 and will be removed in a future version. Please upgrade to @bentoml.service().
30 svc = bentoml.Service("house_price_predictor", runners=[model_runner])
312025-03-04T17:56:58-0500 [INFO] [cli] Environ for worker 0: set CPU thread count to 16
322025-03-04T17:56:58-0500 [INFO] [cli] Prometheus metrics for HTTP BentoServer from "model_service_v1.py" can be accessed at http://localhost:3000/metrics.
332025-03-04T17:56:59-0500 [INFO] [cli] Starting production HTTP BentoServer from "model_service_v1.py" listening on http://0.0.0.0:3000 (Press CTRL+C to quit)
342025-03-04T17:57:06-0500 [INFO] [api_server:house_price_predictor:15] 192.168.60.183:45774 (scheme=http,method=GET,path=/,type=,length=) (status=200,type=text/html; charset=utf-8,length=2945) 0.578ms (trace=218283d5547832c34e6ba268d8dbf47f,span=429596d2b591bb43,sampled=0,service.name=house_price_predictor)
352025-03-04T17:57:07-0500 [INFO] [api_server:house_price_predictor:15] 192.168.60.183:45780 (scheme=http,method=GET,path=/static_content/index.css,type=,length=) (status=200,type=text/css; charset=utf-8,length=1127) 10.881ms (trace=eec4e13972950d1dccc7d4385bb2b5e0,span=437ba5041f163403,sampled=0,service.name=house_price_predictor)
362025-03-04T17:57:07-0500 [INFO] [api_server:house_price_predictor:2] 192.168.60.183:45794 (scheme=http,method=GET,path=/static_content/swagger-initializer.js,type=,length=) (status=200,type=text/javascript; charset=utf-8,length=331) 10.296ms (trace=cabc6765ca89380fccc7d4385bb2b248,span=03ec86ece9db6cdb,sampled=0,service.name=house_price_predictor)
372025-03-04T17:57:07-0500 [INFO] [api_server:house_price_predictor:1] 192.168.60.183:45788 (scheme=http,method=GET,path=/static_content/swagger-ui.css,type=,length=) (status=200,type=text/css; charset=utf-8,length=152059) 12.950ms (trace=99ae2d664c29cc40ccc7d4385bb2bc68,span=324fde3f91555ab3,sampled=0,service.name=house_price_predictor)
382025-03-04T17:57:07-0500 [INFO] [api_server:house_price_predictor:7] 192.168.60.183:45810 (scheme=http,method=GET,path=/static_content/swagger-ui-standalone-preset.js,type=,length=) (status=200,type=text/javascript; charset=utf-8,length=230777) 12.003ms (trace=c6cb37a9f7b5a6fbccc7d4385bb2b5a1,span=84f59425694af0de,sampled=0,service.name=house_price_predictor)
392025-03-04T17:57:07-0500 [INFO] [api_server:house_price_predictor:4] 192.168.60.183:45804 (scheme=http,method=GET,path=/static_content/swagger-ui-bundle.js,type=,length=) (status=200,type=text/javascript; charset=utf-8,length=1415333) 18.999ms (trace=dada38ec3c0f8c73ccc7d4385bb2b272,span=0c9efc4dada3c4ae,sampled=0,service.name=house_price_predictor)
402025-03-04T17:57:07-0500 [INFO] [api_server:house_price_predictor:7] 192.168.60.183:45816 (scheme=http,method=GET,path=/docs.json,type=,length=) (status=200,type=application/json,length=5098) 16.176ms (trace=05f74885ffe57bbbf55baa70e9234e3d,span=5a470cb921d4142d,sampled=0,service.name=house_price_predictor)
412025-03-04T17:57:07-0500 [INFO] [api_server:house_price_predictor:8] 192.168.60.183:45830 (scheme=http,method=GET,path=/static_content/favicon-light-32x32.png,type=,length=) (status=200,type=image/png,length=640) 10.184ms (trace=a2268a9955f2bc5af55baa70e92344e0,span=a6e967dd5c102f0a,sampled=0,service.name=house_price_predictor)
422025-03-04T17:57:08-0500 [INFO] [api_server:house_price_predictor:15] 192.168.60.183:45834 (scheme=http,method=GET,path=/docs.json,type=,length=) (status=200,type=application/json,length=5098) 18.135ms (trace=7842cf9d35f7d855222e5d341456be4a,span=5c4870b472d91d3c,sampled=0,service.name=house_price_predictor)
43/root/lab/04-bentoml/bentoml-env/lib/python3.12/site-packages/sklearn/utils/validation.py:2739: UserWarning: X does not have valid feature names, but LinearRegression was fitted with feature names
44 warnings.warn(
452025-03-04T17:57:24-0500 [INFO] [runner:house_price_model:1] _ (scheme=http,method=POST,path=/predict,type=application/octet-stream,length=30) (status=200,type=application/vnd.bentoml.NdarrayContainer,length=8) 6.380ms (trace=4fec2891ae7e347622c1a432ad43e5ba,span=0f24fa0eed75df82,sampled=0,service.name=house_price_model)
462025-03-04T17:57:24-0500 [INFO] [api_server:house_price_predictor:2] 192.168.60.183:41594 (scheme=http,method=POST,path=/predict_house_price,type=application/json,length=45) (status=200,type=application/json,length=37) 141.210ms (tra
47
48root@controlplane ~ ➜
49
50root@controlplane ~ ➜ curl -X POST "http://127.0.0.1:3000/predict_house_price" \
51 -H "Content-Type: application/json" \
52 -d '{"square_footage": 2500, "num_rooms": 5}'
53{"predicted_price":360974.6696035242}
54root@controlplane ~ ➜
55
56
57
58root@controlplane ~/lab/04-bentoml via 🐍 v3.12.3 (bentoml-env) ➜ python3 ./model_train_v2.py
59
60root@controlplane ~/lab/04-bentoml via 🐍 v3.12.3 (bentoml-env) ➜ bentoml models list
61 Tag Module Size Creation Time
62 house_price_model_v2:kukdhzpzjskuvisu bentoml.sklearn 1.56 KiB 2025-03-04 17:59:31
63 house_price_model:zbsn3yhzjockdisu bentoml.sklearn 1.23 KiB 2025-03-04 17:55:35
64
65root@controlplane ~/lab/04-bentoml via 🐍 v3.12.3 (bentoml-env) ➜
66
67
68root@controlplane ~/lab/04-bentoml via 🐍 v3.12.3 (bentoml-env) ➜ bentoml serve ./model_service_v2.py --reload
69/root/lab/04-bentoml/bentoml-env/lib/python3.12/site-packages/bentoml/io.py:7: BentoMLDeprecationWarning: `bentoml.io` is deprecated since BentoML v1.4 and will be removed in a future version. Please upgrade to new style IO types instead.
70 warn_deprecated(
71/root/lab/04-bentoml/bentoml-env/lib/python3.12/site-packages/bentoml/_internal/models/model.py:367: BentoMLDeprecationWarning: `get_runnable` is deprecated since BentoML v1.4 and will be removed in a future version. Use `get_service` instead.
72 self._runnable = self.info.imported_module.get_runnable(self)
73/root/lab/04-bentoml/bentoml-env/lib/python3.12/site-packages/bentoml/_internal/models/model.py:354: BentoMLDeprecationWarning: `Runner` is deprecated since BentoML v1.4 and will be removed in a future version. Please upgrade to new style services.
74 return Runner(
75/root/lab/04-bentoml/model_service_v2.py:10: BentoMLDeprecationWarning: `bentoml.Service` is deprecated since BentoML v1.4 and will be removed in a future version. Please upgrade to @bentoml.service().
76 svc = bentoml.Service("house_price_predictor_v2", runners=[model_runner])
772025-03-04T18:01:21-0500 [INFO] [cli] Environ for worker 0: set CPU thread count to 16
782025-03-04T18:01:21-0500 [INFO] [cli] Prometheus metrics for HTTP BentoServer from "./model_service_v2.py" can be accessed at http://localhost:3000/metrics.
792025-03-04T18:01:21-0500 [INFO] [cli] Starting production HTTP BentoServer from "./model_service_v2.py" listening on http://0.0.0.0:3000 (Press CTRL+C to quit)
80/root/lab/04-bentoml/ben
81
822025-03-04T18:01:51-0500 [INFO] [api_server:house_price_predictor_v2:14] 192.168.60.183:54112 (scheme=http,method=GET,path=/,type=,length=) (status=200,type=text/html; charset=utf-8,length=2945) 0.564ms (trace=98ed80dd6901b2111ad2e3684c05a0e9,span=6f41af487e967bc1,sampled=0,service.name=house_price_predictor_v2)
832025-03-04T18:01:51-0500 [INFO] [api_server:house_price_predictor_v2:11] 192.168.60.183:54124 (scheme=http,method=GET,path=/static_content/index.css,type=,length=) (status=304,type=,length=) 9.394ms (trace=d02dac653c2560c61ad2e3684c05a705,span=690c3c9f09a8a78d,sampled=0,service.name=house_price_predictor_v2)
842025-03-04T18:01:51-0500 [INFO] [api_server:house_price_predictor_v2:10] 192.168.60.183:54126 (scheme=http,method=GET,path=/static_content/swagger-ui-bundle.js,type=,length=) (status=304,type=,length=) 12.498ms (trace=588d665e1a96ffaa1ad2e3684c05a001,span=69709ac1b2c53d7a,sampled=0,service.name=house_price_predictor_v2)
852025-03-04T18:01:51-0500 [INFO] [api_server:house_price_predictor_v2:8] 192.168.60.183:54130 (scheme=http,method=GET,path=/static_content/swagger-ui.css,type=,length=) (status=304,type=,length=) 8.980ms (trace=ecdcd48cc1491f2d1ad2e3684c05a4f0,span=29bb04cdec45bd70,sampled=0,service.name=house_price_predictor_v2)
862025-03-04T18:01:51-0500 [INFO] [api_server:house_price_predictor_v2:10] 192.168.60.183:54136 (scheme=http,method=GET,path=/static_content/swagger-ui-standalone-preset.js,type=,length=) (status=304,type=,length=) 7.883ms (trace=b5a9e05e271450971ad2e3684c05a216,span=564d36c5c3fb549d,sampled=0,service.name=house_price_predictor_v2)
872025-03-04T18:01:51-0500 [INFO] [api_server:house_price_predictor_v2:11] 192.168.60.183:54152 (scheme=http,method=GET,path=/static_content/swagger-initializer.js,type=,length=) (status=304,type=,length=) 1.128ms (trace=bdadbbcc5a1769d01ad2e3684c05a4d3,span=7780ea74b1e9c935,sampled=0,service.name=house_price_predictor_v2)
882025-03-04T18:01:52-0500 [INFO] [api_server:house_price_predictor_v2:14] 192.168.60.183:54168 (scheme=http,method=GET,path=/docs.json,type=,length=) (status=200,type=application/json,length=6272) 16.935ms (trace=2be593136933d8c81ad2e3684c05af36,span=c75e4918fc8c2bcd,sampled=0,service.name=house_price_predictor_v2)
892025-03-04T18:01:52-0500 [INFO] [api_server:house_price_predictor_v2:6] 192.168.60.183:54176 (scheme=http,method=GET,path=/static_content/favicon-light-32x32.png,type=,length=) (status=304,type=,length=) 9.380ms (trace=302248365757193a1ad2e3684c05afde,span=d15966acfcbe8f92,sampled=0,service.name=house_price_predictor_v2)
902025-03-04T18:01:52-0500 [INFO] [api_server:house_price_predictor_v2:10] 192.168.60.183:54190 (scheme=http,method=GET,path=/docs.json,type=,length=) (status=200,type=application/json,length=6272) 14.924ms (trace=54540160dd445b58331313b7d7b4f058,span=6ed0ae37f52808a2,sampled=0,service.name=house_price_predictor_v2)
91
92
93
94root@controlplane ~/lab/04-bentoml via 🐍 v3.12.3 (bentoml-env) ✖ bentoml serve ./model_service_v3.py --reload
95/root/lab/04-bentoml/bentoml-env/lib/python3.12/site-packages/bentoml/io.py:7: BentoMLDeprecationWarning: `bentoml.io` is deprecated since BentoML v1.4 and will be removed in a future version. Please upgrade to new style IO types instead.
96 warn_deprecated(
97/root/lab/04-bentoml/bentoml-env/lib/python3.12/site-packages/bentoml/_internal/models/model.py:367: BentoMLDeprecationWarning: `get_runnable` is deprecated since BentoML v1.4 and will be removed in a future version. Use `get_service` instead.
98 self._runnable = self.info.imported_module.get_runnable(self)
99/root/lab/04-bentoml/bentoml-env/lib/python3.12/site-packages/bentoml/_internal/models/model.py:354: BentoMLDeprecationWarning: `Runner` is deprecated since BentoML v1.4 and will be removed in a future version. Please upgrade to new style services.
100 return Runner(
101/root/lab/04-bentoml/model_service_v3.py:12: BentoMLDeprecationWarning: `bentoml.Service` is deprecated since BentoML v1.4 and will be removed in a future version. Please upgrade to @bentoml.service().
102 svc = bentoml.Service("house_price_predictor", runners=[model_v1_runner, model_v2_runner])
1032025-03-04T18:03:05-0500 [INFO] [cli] Environ for worker 0: set CPU thread count to 16
1042025-03-04T18:03:05-0500 [INFO] [cli] Environ for worker 0: set CPU thread count to 16
1052025-03-04T18:03:05-0500 [INFO] [cli] Prometheus metrics for HTTP BentoServer from "./model_service_v3.py" can be accessed at http://localhost:3000/metrics.
1062025-03-04T18:03:06-0500 [INFO] [cli] Starting production HTTP BentoServer from "./model_service_v3.py" listening on http://0.0.0.0:3000 (Press CTRL+C to quit)
107 warnings.warn(
1082025-03-04T18:03:14-0500 [INFO] [runner:house_price_model_v2:1] _ (scheme=http,method=POST,path=/predict,type=application/octet-stream,length=71) (status=200,type=application/vnd.bentoml.NdarrayContainer,length=8) 6.956ms (trace=822c5d06fa4b91abbc4219b34ff200a3,span=77fcaceb2e048038,sampled=0,service.name=house_price_model_v2)
1092025-03-04T18:03:14-0500 [INFO] [api_server:house_price_predictor:7] 127.0.0.1:39714 (scheme=http,method=POST,path=/predict_house_price_v2,type=application/json,length=206) (status=200,type=application/json,length=40) 13.287ms (trace=822c5d06fa4b91abbc4219b34ff200a3,span=1cfdcd13835fe0cb,sampled=0,service.name=house_price_predictor)
110/root/lab/04-bentoml/bentoml-env/lib/python3.12/site-packages/sklearn/utils/validation.py:2739: UserWarning: X does not have valid feature names, but LinearRegression was fitted with feature names
111 warnings.warn(
1122025-03-04T18:03:30-0500 [INFO] [runner:house_price_model:1] _ (scheme=http,method=POST,path=/predict,type=application/octet-stream,length=30) (status=200,type=application/vnd.bentoml.NdarrayContainer,length=8) 1.691ms (trace=5dc25830ea64469475a069536956f824,span=8135a80507ce9164,sampled=0,service.name=house_price_model)
1132025-03-04T18:03:30-0500 [INFO] [api_server:house_price_predictor:14] 127.0.0.1:49944 (scheme=http,method=POST,path=/predict_house_price_v1,type=application/json,length=40) (status=200,type=application/json,length=40) 126.227ms (trace=5dc25830ea64469475a069536956f824,span=c94cce88f7a5df33,sampled=0,service.name=house_price_predictor)
114/root/lab/04-bentoml/bentoml-env/lib/python3.12/site-packages/sklearn/utils/validation.py:2739: UserWarning: X does not have valid feature names, but LinearRegression was fitted with feature names
115 warnings.warn(
1162025-03-04T18:03:39-0500 [INFO] [runner:house_price_model_v2:1] _ (scheme=http,method=POST,path=/predict,type=application/octet-stream,length=71) (status=200,type=application/vnd.bentoml.NdarrayContainer,length=8) 1.613ms (trace=7a960e1fc28f21a4aa38e6e018db30ad,span=1f78d735ebc041f0,sampled=0,service.name=house_price_model_v2)
1172025-03-04T18:03:39-0500 [INFO] [api_server:house_price_predictor:12] 127.0.0.1:36020 (scheme=http,method=POST,path=/predict_house_price_v2,type=application/json,length=283) (status=200,type=application/json,length=40) 138.923ms (trace=7a960e1fc28f21a4aa38e6e018db30ad,span=f3a6c975024a5938,sampled=0,service.name=house_price_predictor)
118root@controlplane ~ ➜
119
120
121root@controlplane ~ ➜ # Example request for Version 1. You can use any values in the request body.
122
123 curl -X POST "http://127.0.0.1:3000/predict_house_price_v1" \
124 -H "Content-Type: application/json" \
125 -d '{"square_footage": 2500, "num_rooms": 5}'
126{"predicted_price_v1":360974.6696035242}
127root@controlplane ~ ➜ # Example request for Version 2. You can use any values in the request body.
128
129 curl -X POST "http://127.0.0.1:3000/predict_house_price_v2" \
130 -H "Content-Type: application/json" \
131 -d '{
132 "square_footage": 2500,
133 "num_rooms": 5,
134 "num_bathrooms": 3,
135 "house_age": 10,
136 "distance_to_city_center": 8,
137 "has_garage": 1,
138 "has_garden": 1,
139 "crime_rate": 0.2,
140 "avg_school_rating": 8,
141 "country": "Germany"
142 }'
143{"predicted_price_v2":366760.9746143092}
144root@controlplane ~ ➜
Monitoring ML Models
1ML-Model Monitoring
2
3Evidently AI for Data drift detection...
4
5Model monitoring is not one-time setup...it is a continuous process.... start small imrpove on top of it.